Learn to create a Rock Paper Scissors game using Python. This program is a project-based approach for teaching python in a fun way. It intends you to teach basic concepts like the working of loops, setting variables, and more. List of best Python courses for beginners to get a job.
Rock, paper scissor game is similar to a well-known kids game in which players choose a random number of fingers, and based on these numbers they decide whether to choose rock paper or scissor.
Learn from the top Python courses on Educative for coding interviews with the best tutorials. Get a 7-day free trial, read our Educative review.
Rock Paper Scissor is one of the most traditional games that you can easily build in Python. It helps you to develop many basic concepts that are important for kick-starting your Python journey. Learn to Create the Rock Paper and Scissors game. Read Also: How Long Does It Take To Learn Coding?
How to Play Rock Paper Scissors game?
Since this game is computer versus user, so we have the user move and the computer moves.
In Computer move, Computer chooses a random number from a list of three numbers 1, 2, 3, and based on these numbers, the computer chooses one of the Rock, paper, or scissors.
Priority of choices
- Paper has higher priority as compared to Rock.
- Scissor has higher priority than paper but least priority then rock.
- In the same way, rock has a higher priority than scissors but less priority than paper.
In the user move, the user has a priority to select Rock Paper or Scissors.
- If user choice matches with computer one there is a tie the game.
- One who has a priority of choice than the other one wins the game.
Python Implementation of Rock, Paper, Scissors game
Import random and sys, as we require randomly draw one of – rock paper scissor and also want to exit the program on demand of the user. Then we are initializing three variables with a win, loss, and tie, and all set to 0.
import random, sys
print("n tttt ****** Rock Paper Scissors *****")
# Trck win, loss and tie.
win = 0
loss = 0
tie = 0
Loop into the main game and set the exit condition when the user presses q exit() will be called to exit the game. The randit function chooses a random number from 1 2 and 3 based on these three random numbers we are setting computer move as Rock paper and scissor, with if and elif statements.
# Loop the main game.
while True:
print(f"Win: {win}nLoss: {loss}nTie: {tie}")
print("""Enter Your Move:
r - rock
p - paper
s - scissors
q - quit""")
UserMove = input("n Type one of r, p, s or q : ")
if UserMove == 'q':
sys.exit() #Quit the program.
# Display what the computer choice:
randomNumber = random.randint(1, 3)
With if and else conditionals, we are setting the computer to move as per the numerical choices we have.
#Let Computer Choose it's move.
if randomNumber == 1:
computerMove = 'r'
print("Rock")
elif randomNumber == 2:
computerMove = 'p'
print("Paper")
elif randomNumber == 3:
computerMove = 's'
print("Scissors")
We are now setting a situation if there is a tie, which means that if the user and computer moves are the same we are incrementing the tied variable with 1 and no loss or win in the game.
Winning logic is set according to the priority mentioned above. Between rock and scissor, rock has higher priority and so incrementing the win with one.
Similarly, all these priorities are set according to priority rules. If there is a win, the win variable is incremented by 1 and if there is a loose, loose variable is incremented by 1.
#Check Win
if UserMove == computerMove:
print("It is tie:!")
tie += 1
elif UserMove == 'r' and computerMove == 's':
print("You win!")
win += 1
elif UserMove == "p" and computerMove == 'r':
win += 1
print("You win!")
elif UserMove == "s" and computerMove == 'p':
win += 1
print("You win!")
elif UserMove == "r" and computerMove == 'p':
loss += 1
print("You Lose!")
elif UserMove == "p" and computerMove == 's':
loss += 1
print("You Lose!")
elif UserMove == "s" and computerMove == 'r':
loss += 1
print("You Lose!")
Final Code for Rock Paper and Scisssors
import random, sys
print("n tttt ****** Rock Paper Scissors *****")
# Trck win, loss and tie.
win = 0
loss = 0
tie = 0
# Loop the main game.
while True:
print(f"Win: {win}nLoss: {loss}nTie: {tie}")
print("""Enter Your Move:
r - rock
p - paper
s - scissors
q - quit""")
UserMove = input("n Type one of r, p, s or q : ")
if UserMove == 'q':
sys.exit() #Quit the program.
# Display what the computer choice:
randomNumber = random.randint(1, 3)
#Let Computer Choose it's move.
if randomNumber == 1:
computerMove = 'r'
print("Rock")
elif randomNumber == 2:
computerMove = 'p'
print("Paper")
elif randomNumber == 3:
computerMove = 's'
print("Scissors")
#Check Win
if UserMove == computerMove:
print("It is tie:!")
tie += 1
elif UserMove == 'r' and computerMove == 's':
print("You win!")
win += 1
elif UserMove == "p" and computerMove == 'r':
win += 1
print("You win!")
elif UserMove == "s" and computerMove == 'p':
win += 1
print("You win!")
elif UserMove == "r" and computerMove == 'p':
loss += 1
print("You Lose!")
elif UserMove == "p" and computerMove == 's':
loss += 1
print("You Lose!")
elif UserMove == "s" and computerMove == 'r':
loss += 1
print("You Lose!")
OutPut
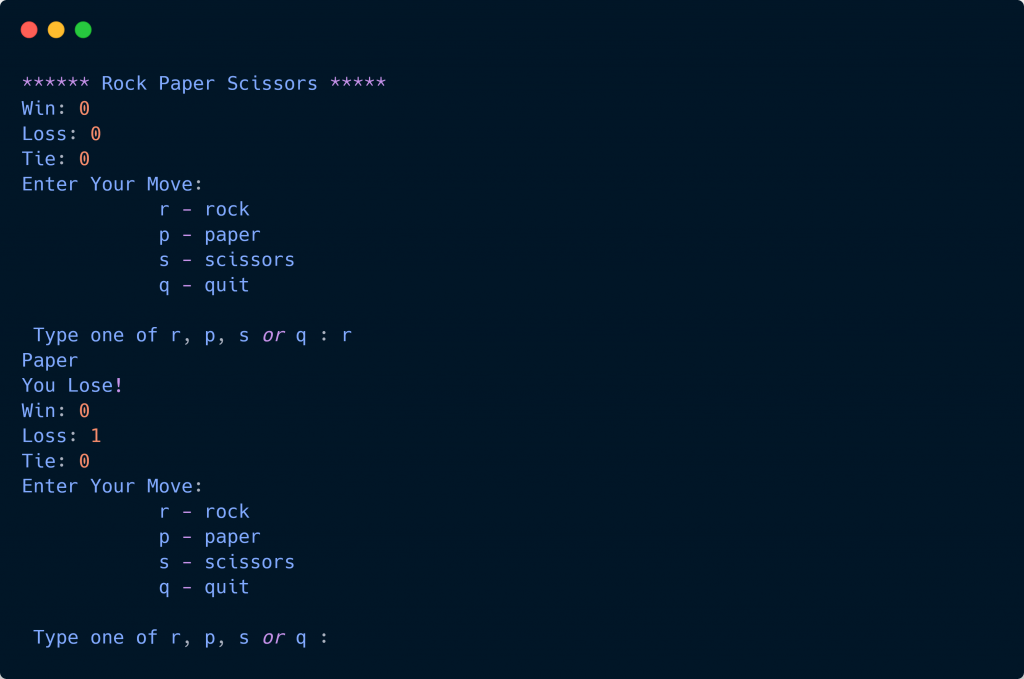
Final Thoughts
This was the implementation of the Rock Paper and Scissors game. You can learn basic concepts here. Learn string operation in Python: Check the best courses for grokking the coding interviews?
Let me know if you have any questions.
Pingback: Is Codecademy Pro Worth It in 2021? [Codeacademy Pro Review]
Pingback: What Is Difference between HTML and HTML5?
Pingback: How Long Does It Take To Learn Coding? The Yuvas