In this article, we will look at 4 ways to check if a string contains a substring in the Python programming language. It is a common way to check in python a string contains a substring and you might encounter this problem in solving any algorithm or doing some operations. How to Learn Python to start a Career?
✨Here’s the detailed course for coding interview that helped people to get job in biggest tech companies around the world like Apple, Google, Tesla, Amazon, JP Morgan, IBM, UNIQLO etc.
👉 Master the Coding Interview: Data Structures + Algorithms 👈
Method 1: Using The in Operator
We have a substring and a string, to check if a substring is found in the string we are using the in operator here. Check the career aspects of Python.
If the substring is found in the string then this statement returns true, otherwise returns false.
The statement substring in string returns True
, if the match is found, else it returns False
.
>>> string = "I am learning Python Programming"
>>> substring = "Python"
>>>
>>> substring in string
True
>>> substring = "Java"
>>> substring in string
False
The in operator is just a shorthand way of writing the method __contains__(key).
If you use string.__contains__(substring), give the same result as the in Operator
>>> string = "I am learning Python Programming"
>>> substring = "Python"
>>>
>>>string.__contains__(substring)
>>>True
Method 2: Using string.find() Method
Another method for checking if a string contains substring is using the find method. It takes substring as input and returns integer. The find method returns the first index of a substring in the final string. But if there is not any match the find method returns -1.
>>> string = "I am learning Python Programming"
>>> substring = "Python"
>>> string.find(substring)
14
>>> substring = "Java"
>>> string.find(substring)
-1
It is better to use the find method if you have to find the index of a substring in a string as well as the existance of the substring. Learn Python from scratch – Best Python courses for beginners.
Method 3: Using Regular Expression (REGEX)
A regular expression (in short REGEX) is a sequence of characters that form the search pattern.
The search method in regex returns an object with a range of indices and word to match.
>>> string = "I am learning Python Programming"
>>> substring = "Python"
>>> search(substring, string)
<re.Match object; span=(14, 20), match='Python'>
The search function of regex returns an object with span and match. The span is the range of index of match string.
>>> search(substring, string).span()
(14, 20)
If the match is not found the search function returns None
>>> print(search("JavaScript", string).span())
None
If you need to find a more complex pattern you can use the REGEX. But for the simple substring, you can avoid using regex, because of its slow speed.
Method 4: Write your function for finding substring in a string
You can write your function for finding substring in a string if you are not allowed to use these built-in methods.
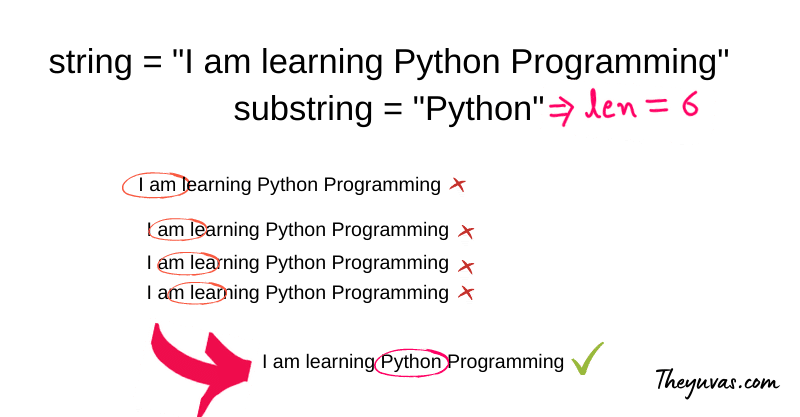
Learn Python from beginner to advanced with 12+ real world professional portfolio projects. Be Job ready with the Python by mastering concepts of OOPs and popular packages.
Here you can check the substring of the same length in the string one by one, if found return the index or return -1.
def findSubstring(string, substring):
M = len(string)
N = len(substring)
# Check all unique strings
for i in range(M - N + 1):
# check for substring
for j in range(N):
if (string[i + j] != substring[j]):
break
if j + 1 == N :
return i
return -1
print(findSubstring(string, substring)) #13
Time Complexity: O(M*N), depends on length of string and substring.
The overall complexity of the function increases with an increase in the length of the string.
Instead, using the index method you can also find the occurrence of a substring in a string if it is clear that a substring is in the string. Otherwise, it returns a value error.
>>> string.index(substring)
13
Phython is one of the most used programming language for solving DSA problems. We’ve listed best platform to practice codig question, free as well as paid options. To come out of this situation you can use try and except statement. Python legendry project: Rock/Paper/Scissors.